Before reading this tutorial you should have knowledge of functions.
A structure is a form of compound data type. It is an aggregate of data items of different data types. It acts as a cluster of variables of different data types. The variables that form the structure are called members. For example, you can have a structure of type employee which stores name, age, qualification, and telephone no and other information about the employee under one data type. A structure can be declared as:-
struct Employee
{
char name[50];
int age;
char quali[70];
int teleno;
char info[80];
};
The keyword struct declares that Employee is a structure. The type name of the structure is Employee. The variables declared are the members of the structure Employee. Every variable of type Employee will contain members name, age, quali, teleno and info. The declaration of the structure is terminated by a semicolon. The data types of the members can be of any type except the type of the structure being defined. The amount of memory need to store a structure type is the total of the memory required by the data types of the members of the structure. The variables of the type Employee can be declared as follows:-
struct Employee emp1; or Employee emp1;
The keyword struct is optional in C++. The variable emp1 is of type Employee. The variables of the structure can be declared along with declaration of the structure. For example,
struct Employee
{
char name[50];
int age;
char quali[70];
int teleno;
char info[80];
}emp1,emp2;
The variables emp1 and emp2 are of type Employee. The members of the structure can be accessed using a dot operator. The general form for accessing the member of a structure is:-
structure_name.member_name
The structure_name is the variable of type structure. The member_name is the name of the member of the structure. For example
emp1.age=20
The variable emp1 is of type Employee and age is the member of the structure Employee. Here is a program which illustrates the working of structures.
#include<iostream>
using namespace std;
struct Employee
{
char name[50];
int age;
char quali[70];
int teleno;
char info[80];
}emp1;
int main ()
{
Employee emp2;
cout << "Enter the name of the employee" << endl;
cin >> emp1.name;
cout << endl << "Enter the age of the employee" << endl;
cin >> emp1.age;
cout << endl << "Enter the qualification of the employee" << endl;
cin >> emp1.quali;
cout << endl << "Enter the telephone no of the employee" << endl;
cin >> emp1.teleno;
cout << endl << "Enter other information about the employee" << endl;
cin >> emp1.info;
emp2=emp1;
cout << endl << "The name of the employee : " << emp2.name << endl;
cout << "The age of the employee : " << emp2.age << endl;
return(0);
}
The result of the program is:-

The statement
struct Employee
{
char name[50];
int age;
char quali[70];
int teleno;
char info[80];
}emp1;
declares a structure of type Employee. The members of employee are name, age, quali, teleno and info. The variable emp1 is of type Employee. The declaration ends with a semicolon. The statement
Employee emp2;
declares a variable emp2 of type employee. Members are accessed by using a dot operator. The statements
cout << "Enter the name of the employee" << endl;
cin >> emp1.name;
make the user to enter the name of the employee. The emp1.name stores the name of the employee. Similarly all the other information is entered by the user. The statement
emp2=emp1;
assigns the information contained in emp1 of type Employee to emp2 of type Employee. Now emp2.name is same as emp1.name and similarly all the information of emp1 is same as emp2.
Arrays of Structures
An array of structures can be declared. For example, if we want to store information about 50 employees of the company then we can declare an array of structure of type Employee. For example,
Employee emp[100];
declares array emp of type Employee. Each variable can be accessed using an index. The index begins with zero like other arrays. For example,
cout << "Name of the first employee" << emp[0].name << endl;
will display the name of the first employee. Similarly emp[0].age contains the age of the first employee.
Passing Structures to Functions
The structures and their members can be passed to functions. The structure and member can act as an argument for the function. Here is a program which shows how structures are passed to functions.
#include<iostream>
using namespace std;
struct Employee
{
char name[50];
int age;
char quali[70];
int teleno;
char info[80];
}emp1;
void display(Employee emp3,char name3[50], int age2);
int main()
{
cout << "Enter the name of the employee" << endl;
cin >> emp1.name;
cout << endl << "Enter the age of the employee" << endl;
cin >> emp1.age;
cout << endl << "Enter the qualification of the employee" << endl;
cin >> emp1.quali;
cout << endl << "Enter the telephone no of the employee" << endl;
cin >> emp1.teleno;
cout << endl << "Enter other information about the employee" << endl;
cin >> emp1.info;
display(emp1,emp1.name,emp1.age);
return(0);
}
void display(Employee emp3, char name3[50],int age3)
{
cout << endl << "The name of the employee : " << name3 << endl;
cout << "The age of the employee : " << age3 << endl;
cout << "The qualification of the employee : " << emp3.quali << endl;
cout << "The telephone no of the employee : " << emp3.teleno << endl;
}
The result of the program is:-

The statement
void display(Employee emp3,char name3[50], int age2);
declares a function whose parameter are emp3 of type Employee, name3 of char type and age2 of type integer. The statement
display(emp1,emp1.name,emp1.age);
make a call to the function display. The arguments are emp1 of type Employee, emp1.name and emp1.age which are members of emp1. The arguments are mapped to the parameters of the function. The information of emp1 is mapped to emp3. The members emp1.name and emp1.age are mapped to name3 and age3 respectively. The statements
cout << endl << "The name of the employee : " << name3 << endl;
cout << "The age of the employee : " << age3 << endl;
cout << "The qualification of the employee : " << emp3.quali << endl;
cout << "The telephone no of the employee : " << emp3.teleno << endl;
display the information of emp1.
Pointers and Structures
There can be pointers to structures. Pointers are used for structures for passing structures by reference and for creating linked lists. The structure pointers can be declared as follows:-
Employee *emp1;
Now emp1 is a pointer to the type Employee. The members of the structure using a pointer are accessed using an arrow operator. The '->' is called an arrow operator which consists of minus sign followed by a greater than operator. The members are accessed using arrow operator in the same way as they are accessed using a dot operator. Here is a program which illustrates the working of pointers and structures.
#include<iostream>
using namespace std;
struct Employee
{
char name[50];
int age;
char quali[70];
int teleno;
char info[80];
}emp1;
void display(Employee *emp3);
int main()
{
cout << "Enter the name of the employee" << endl;
cin >> emp1.name;
cout << endl << "Enter the age of the employee" << endl;
cin >> emp1.age;
cout << endl << "Enter the qualification of the employee" << endl;
cin >> emp1.quali;
cout << endl << "Enter the telephone no of the employee" << endl;
cin >> emp1.teleno;
cout << endl << "Enter other information about the employee" << endl;
cin >> emp1.info;
display(&emp1);
cout << endl << "The modified age of the Employee : " << emp1.age << endl;
cout << " The modified telephone no of the Employee : " << emp1.teleno << endl;
return(0);
}
void display(Employee *emp3)
{
emp3->age=emp3->age+10;
emp3->teleno=3299574;
}
The result of the program is:-
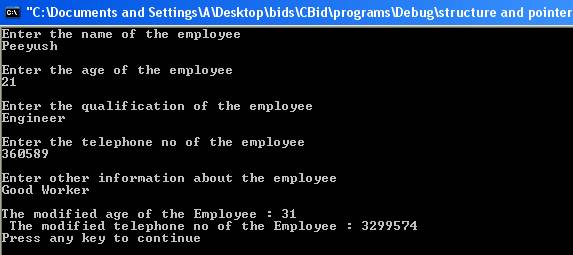
The statement
void display(Employee *emp3);
declares a function whose parameter is a pointer of type Employee. The statement
display(&emp1);
passes the address of emp1 to the function. In the function the statements
emp3->age=emp3->age+10;
emp3->teleno=3299574;
modify the values of the member age and telephone of emp3. The member age and teleno are accessed using an arrow operator. The age is incremented by 10 and telephone no is changed. The statements
cout << endl << "The modified age of the Employee : " << emp1.age << endl;
cout << " The modified telephone no of the Employee : " << emp1.teleno <<
print the modified age and telephone of the employee. The function alters the arguments passed as this pass by reference.
No comments:
Post a Comment